Grunt.js
Grunt.js is the JasvaScript task runner built for high automation of repetitive task like minification, compilation, unit testing, linting, etc.
This article contains several maulas for Grunt usages in Odyssey system about following topics:
Grunt setup and Sass compiler
Images project already contains configured Grunt poject but you need to install few things in order to use it.
Step 01: Install Node.js
Grunt and Grunt plugins are installed and managed via npm, the Node.js package manager. Download and install latest stable version avialabele on Node.js official website in order to run Grunt task on your computer.
Step 02: Install Ruby
Before you start using Sass you will need to install Ruby. The fastest way to get Ruby on your Windows computer is to use Ruby Installer. It's a single-click installer that will get everything set up for you super fast. The installer will also install a Ruby command line powershell application that will let you use the Ruby libraries.
Installation process is straight forward, but you need to check the “Add Ruby executables to your PATH" opton as showen on the image.
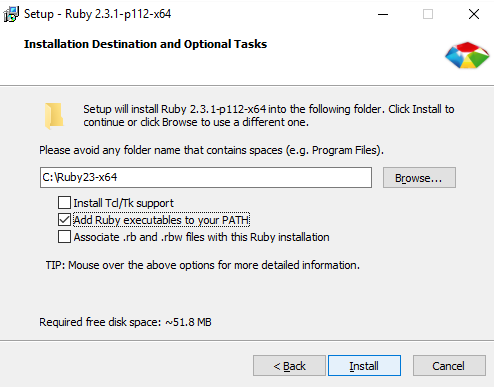
Step 03: Install Sass
Ruby uses Gems to manage its various packages of code like Sass. In your Command Prompt window type:
gem install sass
This will install Sass and any dependencies for you.
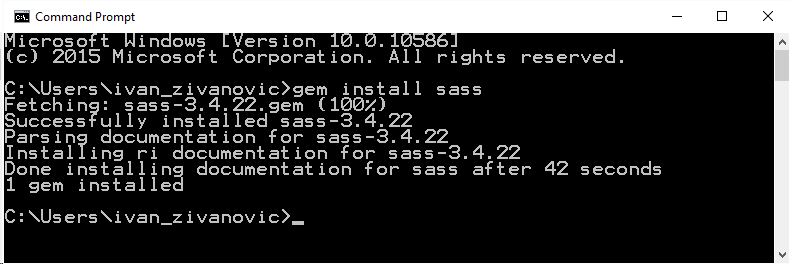
Step 04: Use "Task Runner Explorer" (TRE) in Visual Studio
If this is your first time using Grunt, you will need to show TRE in Visual Studio. To do that go to View > Other Windows > Task Runner Explorer, or simply pres Ctrl+Q and start typing "Task Runner Explorer" and it will appear as the only option.
In order to compile SCSS placed in Images project, select "Images" from the top left select list in the TRE window. After all task are listed, scroll down to the bottom, find "watch:watchScss" task and run it. This will start selected process and it will write "Waiting..." in TRE console on the right.
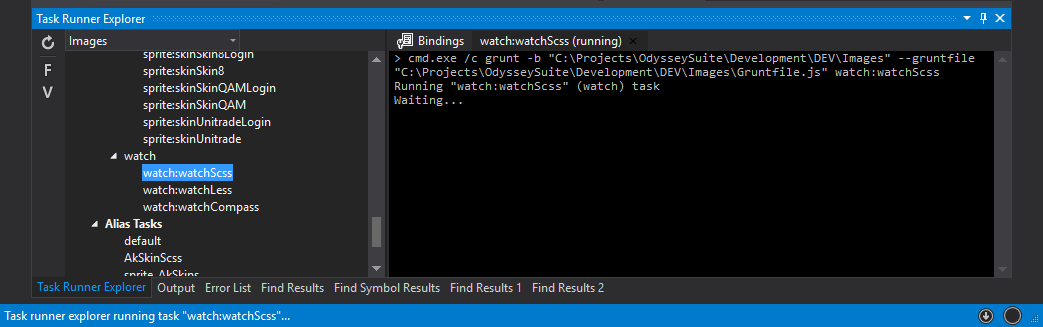
If you are using server workspace CSS file(s) will not be check out automatically, which will cause task failure. Select targeted CSS files, right clik on selection and choose "Check Out for Edit..." option. In prompted window select "Check Out - Prevent other users from checking out and checking in" as the Lock type.
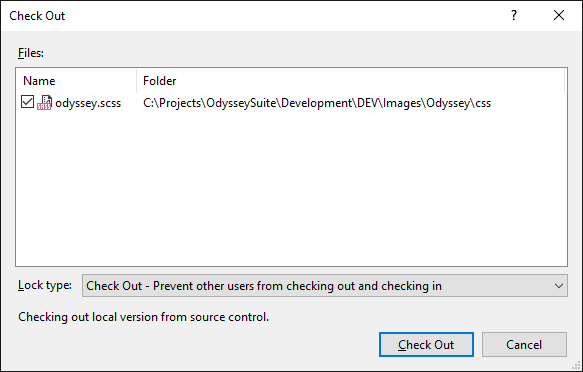
New Grunt project
Existing Grunt porjects will cover all curent development needs, but if you need to start a new project at some point you can do it following this simple steps.
Step 01: Installing the CLI
In order to get started, you'll want to install Grunt's command line interface (CLI) globally. Run your Command Prompt as Administrator and copy this command:
npm install -g grunt-cli
This will put the grunt command in your system path, allowing it to be run from any directory. Note that installing grunt-cli does not install the Grunt task runner! The job of the Grunt CLI is simple: run the version of Grunt which has been installed next to a Gruntfile. This allows multiple versions of Grunt to be installed on the same machine simultaneously.
Step 02: Create package.json file
The package.json file should be placed in the root of your project. This file defines data about the project such as the project name, version and author. The package.json file is also responsible for managing dependencies. The devDependencies property defines the different packages that are needed for your application.
Copy this code to package.json file in order to make a clean start and we'll install Grunt in next step.
{
"name": "project-name",
"version": "0.1.0",
"author": "Quantum - AutoMARKET",
"devDependencies": {
}
}
Step 03: Installing Grunt
Now that we got new project, we can add some modules to it. Grunt and it's plugins are npm packages that needs to be installed so we can use them. Open Command Prompt and navigate to project root directory. Copy this command to install Grunt module and add it as the develoment dependencie.
npm install grunt --save-dev
This will update package.json file adding grunt in "devDependencies" providing other team members whit the instruction for setting things up in their environment easily. During the istallation some errors will be occured, but that does not prevent process from completeing successfuly. Those errors are ecountered because we have not all parameters needed for node project, but we are not deloping node app this is just fine.
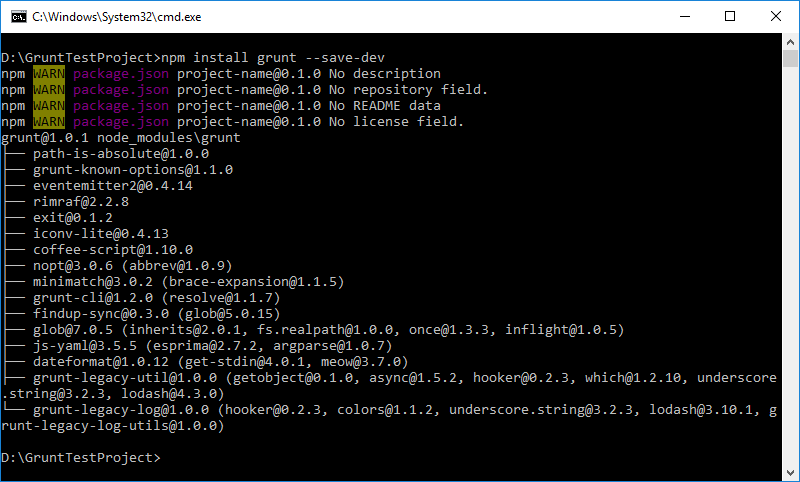
We will add one more moudel for start just have smoething to work with. In previous cahpter you can find instructions for installing Ruby adn Sass to your computer. Those are required for this module to work. To install Sass as the grunt module smiply copy this command to Command Prompt (new project's root should already be locaated):
npm install grunt-contrib-sass --save-dev
Step 04: Create gruntfile.js
The Gruntfile.js or Gruntfile.coffee file is a valid JavaScript or CoffeeScript file that belongs in the root directory of your project, next to the package.json file, and should be committed with your project source.
A Gruntfile is comprised of the following parts:
- The "wrapper" function
- Project and task configuration
- Loading Grunt plugins and tasks
- Custom tasks
This example shows the gruntfile script with the minimum code needed just to compile scss file to css.
// The "wrapper" function
module.exports = function (grunt) {
// Project and task configuration
grunt.initConfig({
// TASKS - compile scss to css
sass: {
dist: {
options: {
style: 'expanded'
},
files: {
'Content/css/master.css': 'Content/scss/master.scss'
}
},
prod: {
options: {
style: 'compressed'
},
files: {
'Content/css/master.min.css': 'Content/scss/master.scss'
}
}
},
}); // END - Project and task configuration
// Loading Grunt plugins and tasks
grunt.loadNpmTasks('grunt-contrib-sass'); // compile scss file to css
// Custom tasks (default task must exist)
grunt.registerTask('default', ['sass']);
}; // END - The "wrapper" function
Step 05: Running Grunt from Command Prompt
In previous chapter we run the grunt tasks from the Visual Studio using Task Runner Explorer, but can be done from the Command Prompt as well. Simply navigate to your project's root directory and type "grunt". When this command is executed "default" task will be triggered.
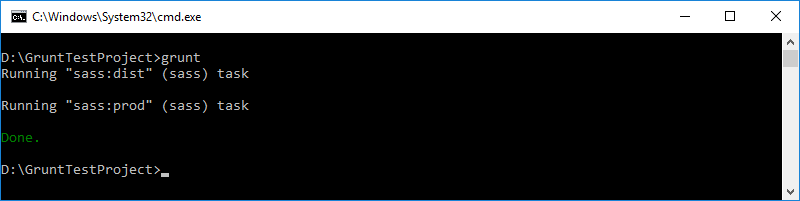